What Are Chrome Extension APIs?
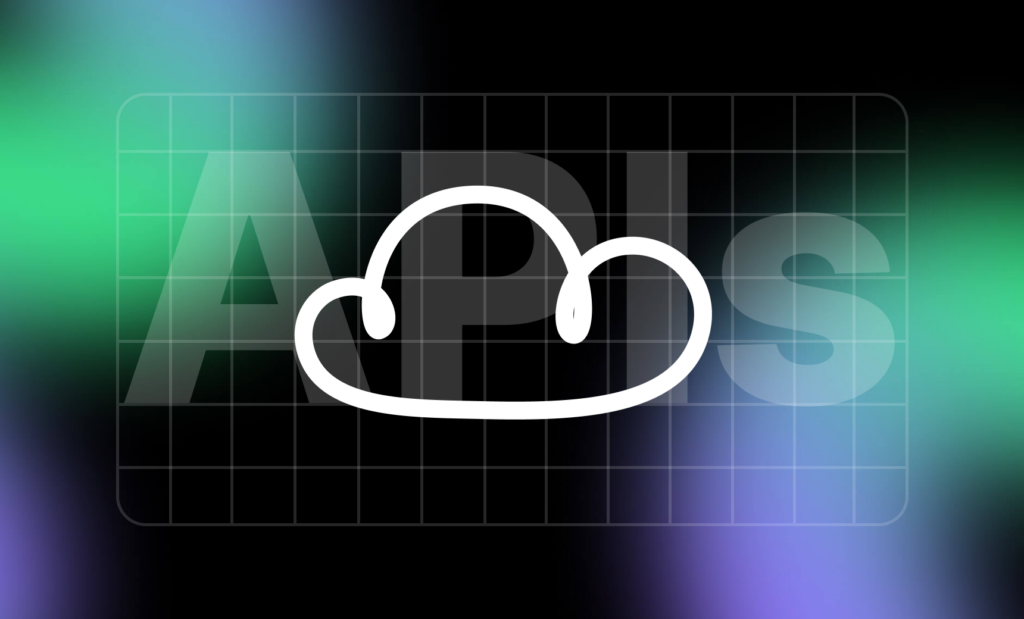
Chrome Extension APIs are a set of application programming interfaces provided by Google for developers to create and enhance browser extensions. These APIs allow interaction with the browser and its components, enabling the addition of new features and improving the user interface.
Action API
What Is the Action API?
The Action API enables developers to add buttons with icons, popups, and badge notifications to the browser’s toolbar. This API allows for quick access to extension features and improves user interaction.
Key Features of the Action API
- Icons and Badges: You can add icons to the toolbar and change them depending on the extension’s state.
- Popups: You can create popups that appear when clicking the extension’s icon.
- Badge Notifications: You can display notifications and change the badge icon based on events.
Example of Using the Action API
// Set the extension icon
chrome.action.setIcon({ path: "icon.png" });
// Add a popup
chrome.action.setPopup({ popup: "popup.html" });
// Set a badge notification
chrome.action.setBadgeText({ text: "5" });
chrome.action.setBadgeBackgroundColor({ color: "#FF0000" });
How to Use the Action API
1. Add API Permissions in the extension manifest
{
"name": "My Extension",
"version": "1.0",
"manifest_version": 3,
"permissions": ["action"],
"action": {
"default_icon": "icon.png",
"default_popup": "popup.html"
}
}
2. Create an Icon and Popup: Place the icon in the extension folder and create a popup.html
file for the popup.
3. Use the API in the extension code to manage icons and popups.
Scripting API
What Is the Scripting API?
The Scripting API provides capabilities for dynamically executing scripts on web pages. With this API, you can add, modify, or remove content from pages and execute JavaScript code in the context of web pages.
Key Features of the Scripting API
- Executing Scripts: Allows the execution of JavaScript code on web pages.
- Code Injection: You can inject code into pages to change their content or add new features.
- DOM Manipulation: You can modify the structure and content of web pages.
Example of Using the Scripting API
// Inject code into the current page
chrome.scripting.executeScript({
target: { tabId: activeTabId },
func: () => {
document.body.style.backgroundColor = 'red';
}
});
// Inject a script from a file
chrome.scripting.executeScript({
target: { tabId: activeTabId },
files: ['content-script.js']
});
How to Use the Scripting API
1. Add API Permissions in the extension manifest
{
"name": "My Extension",
"version": "1.0",
"manifest_version": 3,
"permissions": ["scripting"]
}
2. Create a Content Script: Place JavaScript files in the extension folder that will be injected into web pages.
3. Use the API in the extension code to execute scripts on pages.
SidePanel API
What Is the SidePanel API?
The SidePanel API allows developers to create side panels in the Chrome browser. These panels can contain arbitrary content and provide users with additional information or features related to the current web page.
Key Features of the SidePanel API
- Creating Side Panels: You can add side panels that will appear next to the main web page content.
- Dynamic Content Update: Panels can be updated based on changes to the web page or user actions.
- Interactive Elements: You can add forms, buttons, and other interactive elements to the panels.
Example of Using the SidePanel API
// Add a side panel
chrome.sidePanel.setPanel({
panel: "sidepanel.html",
title: "My Side Panel"
});
// Set content for the panel
chrome.sidePanel.onShown.addListener(() => {
document.getElementById('content').innerHTML = 'Hello, this is the side panel!';
});
How to Use the SidePanel API
1. Add API Permissions in the extension manifest
{
"name": "My Extension",
"version": "1.0",
"manifest_version": 3,
"permissions": ["sidePanel"]
}
2. Create an HTML File for the Panel: Place a sidepanel.html
file in the extension folder and write the HTML code for the panel.
3. Use the API in the extension code to add and manage side panels.
Working with Chrome Extension APIs like Action, Scripting, and SidePanel opens up extensive possibilities for developers to create functional and useful tools. Understanding these APIs and using them effectively can significantly enhance user experience and extend browser functionality. Creating and integrating these features requires careful planning and testing to ensure their proper and effective operation.
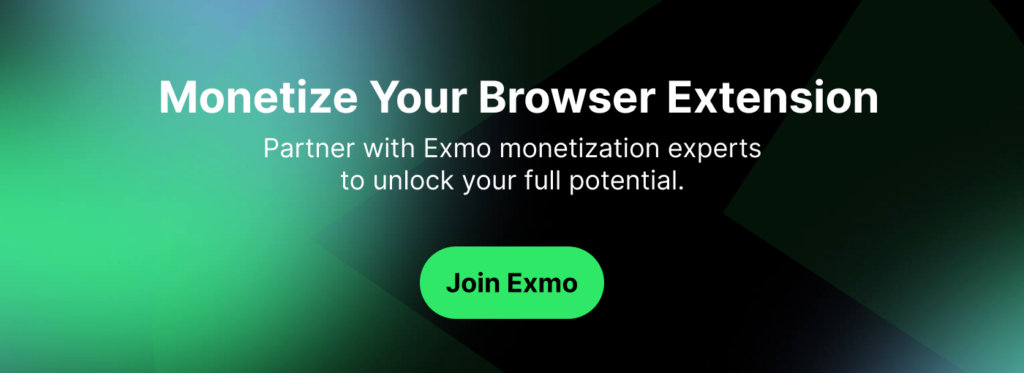
FAQ
What are Chrome Extension APIs?
Chrome Extension APIs are a set of tools provided by Google that allow developers to build and enhance browser extensions. These APIs enable extensions to interact with the browser and web pages, offering capabilities like adding toolbar buttons, executing scripts on pages, and creating custom side panels.
What is the Action API in Chrome Extensions?
The Action API allows developers to add buttons with icons, popups, and badges to the browser’s toolbar. It facilitates quick access to extension features and can display notifications directly within the browser interface.
What is the Scripting API?
The Scripting API allows you to inject and execute JavaScript code on web pages dynamically. It is useful for modifying page content, adding features, or automating interactions on web pages.
How do I implement the Scripting API?
Include the Scripting API in your extension’s manifest file under "permissions"
, and use chrome.scripting.executeScript
in your code to inject JavaScript into web pages. You can either inject code directly or run a script from a file.
Can I use the Scripting API to change the content of any web page?
Yes, but you must respect the content and functionality of the web page, adhere to Chrome Web Store policies, and ensure that your modifications do not disrupt the user experience. Always get user permission if the script significantly alters the page.