Replace blocking web request listeners: Migrate Manifest v2 to v3
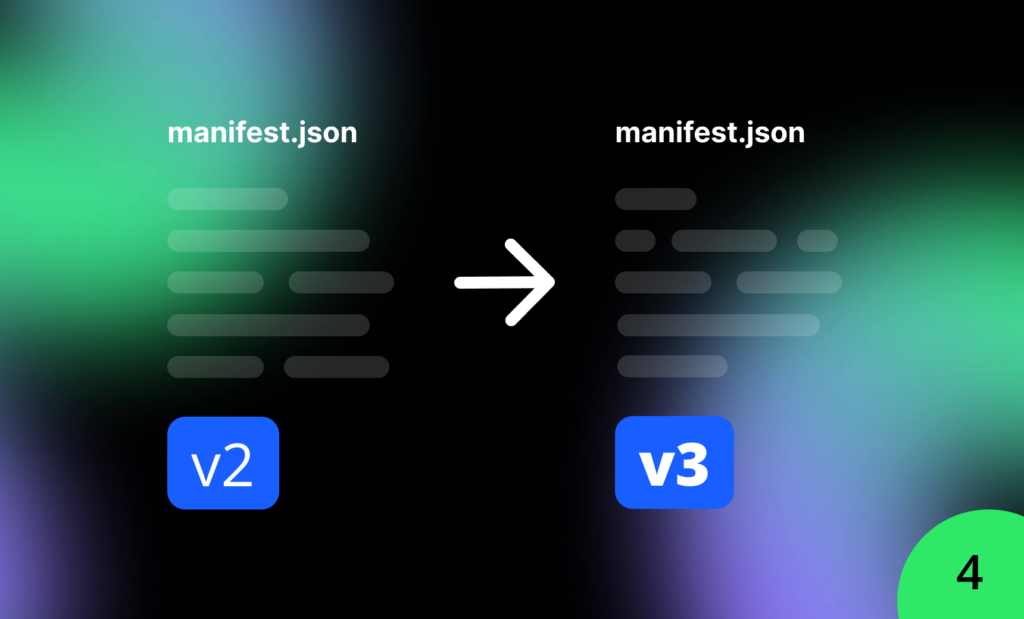
Ensure a seamless transition from Manifest V2 to V3 for your Chrome extension with our comprehensive guide. Learn how to replace blocking web request listeners with the declarativeNetRequest API, leveraging Manifest V3’s enhanced network request management for improved security and performance. Keep your extension functional and up-to-date with the latest standards as you navigate this migration process. Created by Exmo – a monetization platform for browser extensions.
Previos posts:
- Chrome Extension Migrate Manifest v3 from v2
- Migrate to a service worker: Manifest v2 to v3
- Update your code: Chrome Extension Migrate Manifest v3
Manifest V3 brings significant changes to how extensions handle network requests modification. Instead of intercepting and altering network requests dynamically using chrome.webRequest
, Manifest V3 introduces a new approach. Extensions now define rules that specify actions to take when certain conditions are met, using the Declarative Net Request API.
The Declarative Net Request API differs substantially from the Web Request API. Rather than simply replacing one function call with another, the migration process involves rethinking your code in terms of different use cases. This section outlines the steps to achieve this transition.
In Manifest V2, blocking web requests could severely impact both extension performance and the performance of the pages they interact with. The webRequest namespace supports nine potentially blocking events, each of which can have an unlimited number of event handlers. Additionally, multiple extensions could potentially block the same web page, leading to invasive permissions requirements. Manifest V3 addresses these issues by replacing callbacks with declarative rules.
This section focuses on converting blocking web requests, utilized in Manifest V2, to declarative net requests, employed in Manifest V3. It is the second of three sections discussing changes required for code outside the extension service worker. The other sections cover general code updates necessary for migrating to Manifest V3 and enhancing security measures.
Updating Permissions in the Manifest
To ensure compatibility with Manifest V3, you need to make adjustments to the “permissions” field in your manifest.json file. Follow these steps:
- Remove the “
webRequest
” permission if it’s no longer necessary for observing network requests. - Transfer Match Patterns from the “
permissions
” field to the “host_permissions
” field.
Additionally, you may need to add other permissions depending on your specific use case. Each permission is described alongside the corresponding use case it supports.
Creating Declarative Net Request Rules
To create declarative net request rules, you’ll need to include a “declarative_net_request
” object in your manifest.json. Within this block, include an array of “rule_resource
” objects, which point to a rule file. The rule file should contain an array of objects specifying actions and the conditions under which these actions are triggered.
Note: The declarativeNetRequest
API offers extensive functionality, beyond what can be summarized here. Below are a few truncated examples showcasing its use cases. For a comprehensive guide on leveraging the declarativeNetRequest
API, refer to its reference documentation.
Common Use Cases
Below are some common scenarios illustrating the application of declarative net requests. While these examples provide a brief overview, for detailed information on utilizing the declarativeNetRequest
API, refer to its reference documentation under chrome.declarativeNetRequest.
Block a Single URL
In Manifest V2, blocking specific web requests was achieved through the onBeforeRequest
event in the background script.
Manifest V2 Background Script
chrome.webRequest.onBeforeRequest.addListener(
(e) => {
return { cancel: true };
},
{ urls: ["https://www.example.com/*"] },
["blocking"]
);
For Manifest V3, create a new rule in a rule file using the “block
” action type. Note the “condition
” object in the example rule. Its “urlFilter
” replaces the urls option passed to the webRequest
listener. The “resourceTypes
” array specifies the type of resources to block. The example below blocks only the main HTML page, but you could tailor it to, for instance, block only fonts.
Manifest V3 Rule File
[
{
"id": 1,
"priority": 1,
"action": {
"type": "block"
},
"condition": {
"urlFilter": "||example.com",
"resourceTypes": ["main_frame"]
}
}
]
To enable this functionality, update the extension’s permissions. In the manifest.json, replace the “webRequestBlocking
” permission with the “declarativeNetRequest
” permission. The rule file specifies the host or hosts to which a declarative net request applies.
If you want to try this, the code below is available in our samples repo.
Manifest V2
"permissions": [
"webRequestBlocking",
"https://*.example.com/*"
]
Manifest V3
"permissions": [
"declarativeNetRequest",
]
Redirect Multiple URLs
In Manifest V2, redirecting web requests was often done using the BeforeRequest
event.
Manifest V2 Background Script
chrome.webRequest.onBeforeRequest.addListener((e) => {
return { redirectUrl: "https://developer.chrome.com/docs/extensions/mv3/intro/" };
}, {
urls: [
"https://developer.chrome.com/docs/extensions/mv2/"
]
},
["blocking"]
);
For Manifest V3, employ the “redirect
” action type. Similar to before, “urlFilter
” replaces the url option passed to the webRequest
listener. In this example, the rule file’s “action
” object contains a “redirect
” field specifying the URL to return instead of the filtered URL.
Manifest V3 Rule File
[
{
"id": 1,
"priority": 1,
"action": {
"type": "redirect",
"redirect": {
"url": "https://developer.chrome.com/docs/extensions/mv3/intro/"
}
},
"condition": {
"urlFilter": "https://developer.chrome.com/docs/extensions/mv2/",
"resourceTypes": ["main_frame"]
}
}
]
To implement this, update the extension’s permissions. Replace the “webRequestBlocking
” permission with the “declarativeNetRequest
” permission in the manifest.json. The URLs are moved from the manifest.json to a rule file. Redirecting also requires the “declarativeNetRequestWithHostAccess
” permission in addition to the host permission.
If you want to try this, the code below is available in our samples repo.
Manifest V2
"permissions": [
"webRequestBlocking",
"https://developer.chrome.com/docs/extensions/*",
"https://developer.chrome.com/docs/extensions/reference"
]
Manifest V3
"permissions": [
"declarativeNetRequestWithHostAccess"
],
"host_permissions": [
"https://developer.chrome.com/*"
]
Block Cookies
In Manifest V2, blocking cookies involved intercepting web request headers before sending them and removing specific ones.
Manifest V2 Background Script
chrome.webRequest.onBeforeSendHeaders.addListener(
function(details) {
removeHeader(details.requestHeaders, 'cookie');
return {requestHeaders: details.requestHeaders};
},
// filters
{urls: ['https://*/*', 'http://*/*']},
// extraInfoSpec
['blocking', 'requestHeaders', 'extraHeaders']);
In Manifest V3, achieve this with a rule in a rule file using the “modifyHeaders
” action type. The rule file specifies an array of “requestHeaders
” objects indicating the headers to modify and how to modify them. The “condition
” object contains only a “resourceTypes
” array, supporting the same values as previous examples.
If you want to try this, the code below is available in our samples repo.
Manifest V3 Rule File
[
{
"id": 1,
"priority": 1,
"action": {
"type": "modifyHeaders",
"requestHeaders": [
{
"header": "cookie",
"operation": "remove"
}
]
},
"condition": {
"urlFilter": "|*?no-cookies=1",
"resourceTypes": ["main_frame"]
}
}
]
This scenario also necessitates updates to the extension’s permissions. Replace the “webRequestBlocking
” permission with the “declarativeNetRequest
” permission in the manifest.json.
Manifest V2
"permissions": [
"webRequest",
"webRequestBlocking",
"https://*/*",
"http://*/*"
],
Manifest V3
"permissions": [
"declarativeNetRequest",
],
"host_permissions": [
"
Continue reading:
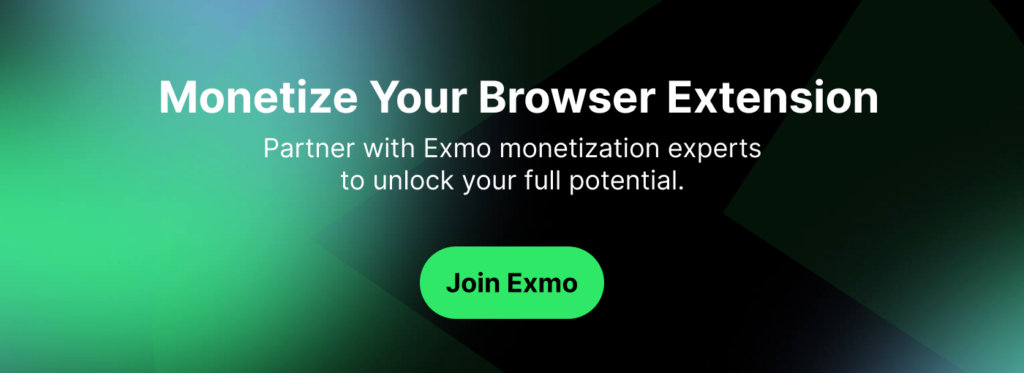
FAQ for Migrate Manifest V2 to V3
Why is it essential to migrate Manifest V2 to V3 for my Chrome extension?
Manifest V3 introduces significant changes to enhance extension security, performance, and maintainability. It is essential to migrate to stay compliant with Chrome’s latest standards and ensure your extension’s continued functionality.
What are blocking web request listeners, and why do they need to be replaced?
Blocking web request listeners were commonly used in Manifest V2 to intercept and modify network requests dynamically. However, they could impact extension and webpage performance negatively. In Manifest V3, blocking web request listeners are replaced with declarative rules provided by the declarativeNetRequest
API, offering better performance and security.
How does the Declarative Net Request API differ from the Web Request API used in Manifest V2?
The Declarative Net Request API, introduced in Manifest V3, defines rules specifying actions to take when certain conditions are met, whereas the Web Request API used in Manifest V2 allowed extensions to intercept and modify network requests dynamically. Declarative rules are more efficient and secure compared to the dynamic nature of the Web Request API.
How can I test the functionality of my extension after migrating to Manifest V3?
You can test the functionality of your extension by loading it in Chrome with the updated manifest file and checking if it behaves as expected. It’s also recommended to review Chrome’s developer documentation and debugging tools for further testing and troubleshooting.
Are there any compatibility considerations or potential issues I should be aware of during the migration process?
While migrating to Manifest V3, ensure compatibility with other Chrome APIs and extensions that your extension interacts with. Additionally, be mindful of any deprecated features or APIs that may impact your extension’s functionality.
Where can I find additional resources and support for migrating my extension to Manifest V3?
You can refer to Chrome’s official developer documentation, community forums, and online tutorials for comprehensive guidance on migrating to Manifest V3. Additionally, seeking assistance from experienced developers or extension development communities can provide valuable insights and support throughout the migration process.