How to Create Chrome Extension Manifest v3
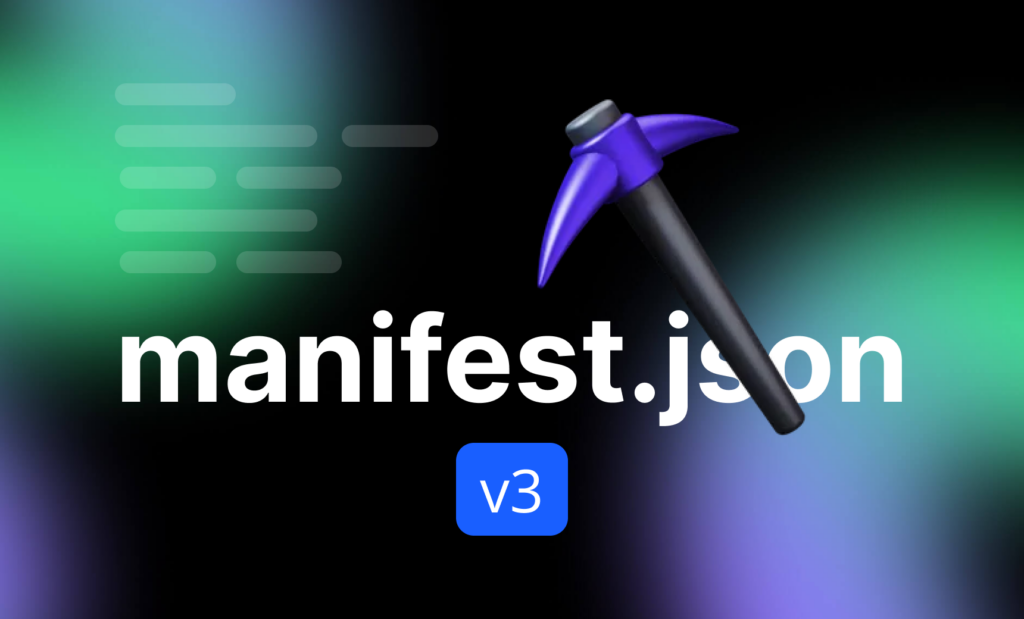
Chrome extensions are small software programs that customize the browsing experience for users of Google Chrome. They can be created by anyone with knowledge of web development, and they can be used to add functionality to the Chrome browser. One of the key components of creating a Chrome extension is the manifest file. Learn how to create a Chrome extension manifest v3 with our comprehensive guide, covering essential elements like permissions, background scripts, content scripts, and more.
The manifest file is a JSON file that contains important information about the extension, such as its name, version, and permissions. It also specifies the files that make up the extension and defines its functionality. Understanding how to create a manifest file is essential for anyone looking to create a Chrome extension.
Creating a manifest file involves specifying the various components of the extension, such as its background scripts, content scripts, and user interface. It also involves defining the permissions that the extension requires, such as access to the user’s browsing history or the ability to modify web pages. By following the proper format and including all the necessary information, developers can create powerful and useful Chrome extensions that enhance the browsing experience for millions of users.
Understanding Manifest Files
A Chrome extension manifest file is a JSON file that contains important information about the extension, such as its name, version, permissions, and background scripts. This file is required for the extension to run properly and is used by the Chrome Web Store to display information about the extension to users.
The manifest file is structured as a key-value pair, with each key representing a specific piece of information about the extension. Some of the most important keys include:
name
: The name of the extension as it will appear in the Chrome Web Store and in the user’s browser.description
: A brief description of the extension and its functionality.version
: The version number of the extension.permissions
: A list of permissions that the extension requires to function properly, such as access to the user’s browsing history or the ability to interact with other extensions.content_scripts
: A list of scripts that the extension will inject into web pages.background
: A script that runs in the background of the extension and can perform tasks such as listening for events or making network requests.
Manifest files are an important part of developing a Chrome extension, as they provide the necessary information for the extension to function properly and be displayed in the Chrome Web Store. By understanding the structure and content of a manifest file, developers can create powerful and functional extensions that enhance the browsing experience for users.
Setting Up the Development Environment
Installing Required Tools
Before creating a Chrome extension manifest, the developer needs to install a few tools. The first tool is Google Chrome, which is the browser that the extension will be developed for. The second tool is a text editor or Integrated Development Environment (IDE) such as Visual Studio Code or Sublime Text. These tools are necessary for writing and testing the code.
Another tool that is required is the Chrome Web Store Developer Dashboard. This tool is used to publish and manage the extension on the Chrome Web Store. The developer needs to have a Google account to use this tool.
Configuring Your IDE
After installing the required tools, the developer needs to configure the IDE. The first step is to install the Chrome Developer Tools extension. This tool is used to debug and test the extension. The developer also needs to enable Developer Mode in the browser, which allows the installation of unpacked extensions.
The developer needs to create a new folder for the extension and add a manifest.json file to the folder. This file contains the metadata for the extension, including the name, version, and permissions. The developer can also add other files such as HTML, CSS, and JavaScript files to the folder.
Once the files are added, the developer can load the extension in the browser by clicking on the Load unpacked button in the Extensions page. The developer can also use the Chrome Developer Tools to debug and test the extension.
In summary, setting up the development environment for creating a Chrome extension manifest requires installing the required tools and configuring the IDE. The developer needs to have Google Chrome, a text editor or IDE, and the Chrome Web Store Developer Dashboard. The developer also needs to configure the IDE by installing the Chrome Developer Tools extension, enabling Developer Mode in the browser, creating a new folder for the extension, and adding a manifest.json file to the folder.
Creating the Chrome Extension Manifest v3 File
To create a Chrome extension, the first step is to create a manifest file. This file provides essential information about the extension to the Chrome browser, such as its name, version, and permissions. In this section, we will cover the basic structure of the manifest file and the information it should contain.
Manifest Version
The first line of the manifest file should specify the version of the manifest file format that the extension uses. The current and only supported value is 3. This line should look like this:
{
"manifest_version": 3,
...
}
Basic Extension Metadata
The next section of the manifest file should contain basic metadata about the extension, such as its name, version, and description. Here is an example of how this section should look:
{
"name": "My Extension",
"version": "1.0",
"description": "This is a description of my extension.",
...
}
Other optional metadata fields include author
, homepage_url
, icons
, and default_locale
.
Defining Extension Capabilities
The final section of the manifest file should define the capabilities of the extension. This includes permissions, content scripts, background scripts, and more. Here is an example of how this section might look:
{
...
"permissions": [
"activeTab",
"storage"
],
"content_scripts": [
{
"matches": [
"https://www.google.com/*"
],
"js": [
"content-script.js"
]
}
],
"background": {
"service_worker": "background-script.js"
}
}
This example grants the extension permission to access the active tab and storage, injects a content script on Google search pages, and runs a service worker as the background script.
By following these guidelines, developers can create a well-structured and effective manifest file for their Chrome extension.
Developing Background Scripts
Background scripts are an essential component of a Chrome extension manifest. They run in the background and are responsible for handling events and performing tasks that require no user interaction.
To develop a background script, the developer must specify it in the manifest file. The script must be a JavaScript file that contains the necessary code to handle events and perform tasks. The background script is loaded when the extension is installed and runs continuously in the background until the extension is uninstalled.
The background script can communicate with the extension’s content scripts and other parts of the extension using message-passing APIs. These APIs allow the background script to receive and send messages to other parts of the extension, such as the popup window or options page.
Developers can also use the storage APIs to store and retrieve data from the extension’s local storage. This data can be used to maintain the extension’s state and provide a better user experience.
It is important to note that background scripts should be designed to be lightweight and efficient to minimize the impact on the user’s browsing experience. Developers should avoid performing heavy computations or operations that may slow down the browser.
In summary, developing background scripts is a crucial part of creating a Chrome extension. By following best practices and using the appropriate APIs, developers can create efficient and reliable background scripts that enhance the functionality of their extensions.
Working with Content Scripts
Content scripts are JavaScript files that run in the context of web pages. They allow extensions to interact with the content of the pages, manipulate the DOM, and perform actions based on the web page’s content. Content scripts are specified in the manifest file and are injected into web pages based on URL patterns.
Specifying Content Scripts in the Manifest
To include content scripts in your extension, you need to add the content_scripts
key in your manifest.json
file. Here’s an example:
{
"manifest_version": 3,
"name": "My Extension",
"version": "1.0",
"description": "This is a description of my extension.",
"permissions": [
"activeTab",
"storage"
],
"content_scripts": [
{
"matches": [
"https://www.google.com/*"
],
"js": [
"content-script.js"
],
"css": [
"styles.css"
]
}
],
"background": {
"service_worker": "background-script.js"
}
}
In this example, the content-script.js
script and styles.css
stylesheet are injected into all pages under the google.com
domain.
Writing Content Scripts
Content scripts can access and manipulate the web page’s DOM. Here’s an example of a simple content script:
// content-script.js
// Change the background color of the page
document.body.style.backgroundColor = "lightblue";
// Add a new paragraph to the page
let paragraph = document.createElement("p");
paragraph.textContent = "This is added by the extension!";
document.body.appendChild(paragraph);
This script changes the background color of the web page to light blue and adds a new paragraph with text to the page.
Handling User Interface Elements
Extensions often provide a user interface (UI) element that allows users to interact with the extension. This UI element can be a popup, options page, or browser action button.
Creating a Popup
A popup is a small window that appears when the user clicks on the extension’s icon in the toolbar. To create a popup, you need to specify the action
key in your manifest.json
file and provide the path to an HTML file that defines the popup’s content.
{
"manifest_version": 3,
"name": "My Extension",
"version": "1.0",
"description": "This is a description of my extension.",
"permissions": [
"activeTab",
"storage"
],
"action": {
"default_popup": "popup.html",
"default_icon": {
"48": "icons/icon48.png",
"128": "icons/icon128.png"
}
},
"content_scripts": [
{
"matches": [
"https://www.google.com/*"
],
"js": [
"content-script.js"
],
"css": [
"styles.css"
]
}
],
"background": {
"service_worker": "background-script.js"
}
}
In this example, the default_popup
is set to popup.html
, which is the HTML file for the popup’s content.
Writing Popup HTML and Script
Here’s an example of a simple popup.html
file:
<!DOCTYPE html>
<html>
<head>
<title>My Extension Popup</title>
<style>
body {
font-family: Arial, sans-serif;
}
</style>
</head>
<body>
<h1>Welcome to My Extension</h1>
<button id="myButton">Click Me!</button>
<script src="popup.js"></script>
</body>
</html>
And the corresponding popup.js
file:
// popup.js
document.getElementById('myButton').addEventListener('click', () => {
alert('Button clicked!');
});
This popup includes a button that, when clicked, shows an alert message.
Debugging and Testing Your Extension
Debugging and testing are crucial steps in the development process to ensure your extension works as expected.
Enabling Developer Mode
To test your extension locally, you need to enable Developer Mode in Chrome:
- Open Chrome and navigate to
chrome://extensions/
. - Toggle the “Developer mode” switch in the top right corner.
- Click the “Load unpacked” button and select the folder containing your extension files.

Using Chrome Developer Tools
Once your extension is loaded, you can use Chrome Developer Tools to debug and test your extension:
- Right-click on your extension’s icon in the toolbar and select “Inspect popup” to debug the popup.
- Use the “Background page” link in the Extensions page to inspect and debug background scripts.
- Open the Console tab to see any log messages and errors.
Publishing Your Extension
Once your extension is ready, you can publish it to the Chrome Web Store.
Preparing for Submission
Before submitting your extension, ensure you have:
- A detailed description of your extension.
- High-quality screenshots showing your extension in action.
- An icon in various sizes (16×16, 48×48, 128×128 pixels).
- A zip file of your extension’s files.
Submitting to the Chrome Web Store
- Go to the Chrome Web Store Developer Dashboard and log in with your Google account.
- Click the “Add a new item” button and upload the zip file of your extension.
- Fill out the required fields, such as description, category, and language.
- Upload screenshots and icons.
- Pay the one-time developer registration fee.
- Submit your extension for review.
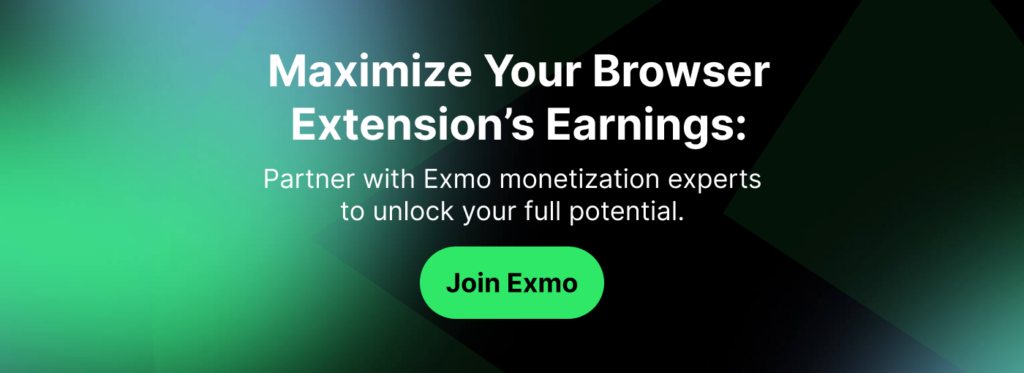
FAQ
What is a Chrome extension manifest file?
A Chrome extension manifest file is a JSON file that contains important metadata about the extension, such as its name, version, permissions, background scripts, content scripts, and other settings. It is essential for defining the extension’s functionality and behavior.
How do I create a Chrome extension manifest v3?
To create a Chrome extension manifest v3, you need to write a JSON file named manifest.json that includes the required fields like manifest_version, name,version
, and permissions
. You can then add other components such as background scripts, content scripts, and action keys based on your extension’s needs.
How can I create a popup for my extension?
To create a popup, you need to define the action
key in your manifest file and provide the path to an HTML file for the popup’s content.
Where can I find more information on creating Chrome extensions?
You can find more information and official documentation on creating Chrome extensions on the Google Chrome Developer Documentation. This site includes detailed guides, API references, and best practices.
Creating a Chrome extension involves understanding the structure and content of the manifest file, developing background and content scripts, handling UI elements, and debugging and testing the extension. By following these guidelines and best practices, developers can create powerful and useful Chrome extensions that enhance the browsing experience for millions of users.